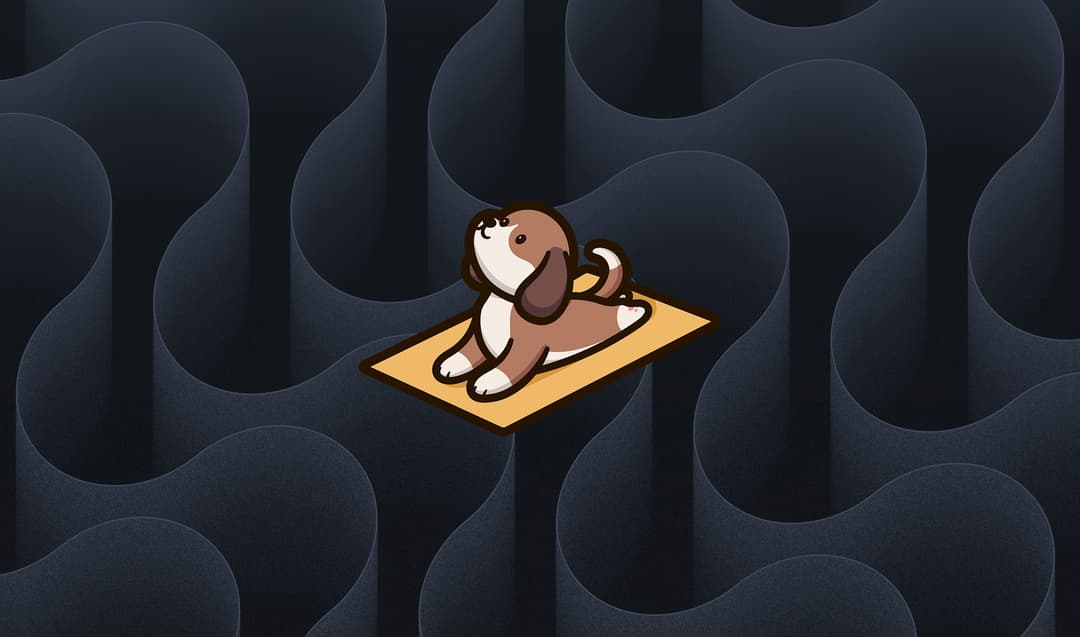
A Web Application Providing Dog Breed Information and Public API
3m 12s
Dog Breed Information Application and Public API
View code on GitHub Visit Live ProjectAs one of my first projects, I undertook the challenge of building a web application that allows users to search for and explore various dog breeds. This project allowed me to learn how API routes work behind the scenes, in a practical way.
This blog post outlines the development process, key features, and some insights into the codebase.
Overview of the Application
The core functionality of the web application revolves around a searchable database of dog breeds. Users can explore information about different breeds, including descriptions, temperaments, popularity, and other attributes. Given that the breed data is static, i chose to store the data in a JSON file, which ensured quick access and simplicity in data management.
Additionally, the application serves as a public API, enabling developers to programmatically fetch the list of dog breeds or details about specific breeds.
Architecture and Design
The project is structured in a clean and modular manner, utilising modern web technologies. A brief overview of the key components:
-
Frontend: Built using React, the frontend provides a responsive and user-friendly interface. Key components include the search bar, breed list, and detailed breed information pages.
-
Backend: The backend serves the static JSON data and handles API requests. It is built using Next.js, which allows for server-side rendering and API route handling.
-
Data Storage: The dog breed data is stored in a
data.json
file. This static file approach simplifies data handling and reduces server load since the data does not change frequently.
Key Features and Code Snippets
Fetching the List of Dog Breeds
To provide a list of all dog breeds, an API endpoint /api/breeds
was created. This endpoint reads the breed data from data.json
and returns a list of breed names.
// app/api/breeds/route.ts
import { NextRequest, NextResponse } from 'next/server';
import dogs from '../data.json';
export async function GET() {
const breedNames = dogs.map(dog => dog.id);
return new NextResponse(JSON.stringify(breedNames), {
status: 200,
headers: {
'Content-Type': 'application/json',
},
});
}
Detailed Breed Information
For users or developers looking for detailed information on a specific breed, the API provides an endpoint /api/dogs
, which accepts an id
parameter to fetch breed-specific details.
// app/api/dogs/route.ts
import { NextRequest, NextResponse } from 'next/server';
import dogs from '../data.json';
export async function GET(request: NextRequest) {
const { searchParams } = new URL(request.url);
const id = searchParams.get('id');
const breed = dogs.find(dog => dog.id === id);
if (breed) {
return new NextResponse(JSON.stringify(breed), {
status: 200,
headers: {
'Content-Type': 'application/json',
},
});
} else {
return new NextResponse(JSON.stringify({ message: 'Breed not found' }), {
status: 404,
headers: {
'Content-Type': 'application/json',
},
});
}
}
Static Site Generation
Next.js's static site generation capabilities are leveraged to pre-render pages at build time. This approach improves performance and SEO, as pages are served as static HTML.
// next.config.mjs
export async function getStaticProps() {
const breeds = dogs.map(dog => dog.id);
return {
props: {
breeds,
},
};
}
Challenges
One of the primary challenges was ensuring efficient data handling, especially since the data is static and extensive. By opting for static site generation and caching data, I managed to keep the application responsive and scalable.
Another aspect was creating a clean and intuitive UI that could handle a large amount of data without overwhelming the user. This was achieved by incorporating thoughtful design principles and using modern frameworks such as React and Tailwind.
Conclusion
Building this web application provided valuable insights into full-stack development. It served as an good portfolio piece, demonstrating skills in frontend and backend development, API design, and data handling. As I continue to refine my skills, projects like this create a solid foundation for creating more complex and dynamic web applications in the future.